Change Facebook Cover or Profile Pic with PHP
I have seen people making separate souvenir page on their websites, where they put some wonderful wallpapers, mini e-card or freewares, something to remember them by. But now times are changing with Facebook trend, so why not add some cool custom made Facebook cover images for your visitors? I am sure it will surely increase some visibility of your website on Facebook.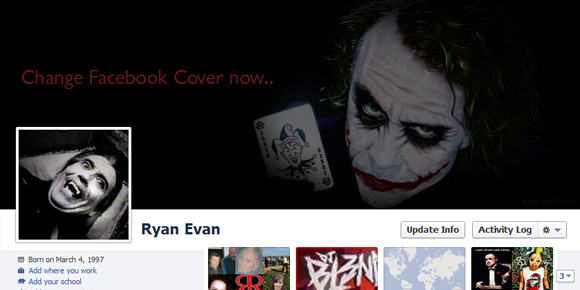
Cover Pictures List
Let's list our images on the page. You can simple use HTML to list your images as shown in code below, or you can keep info in a database table and fetch them, it's upto you. You can also use CSS to make it look interesting. As you can see all image links point to process.php, that is where the real thing will happen.HTML
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
<title>Change Facebook Cover or Profile Pic with PHP Demo</title>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.js"></script>
<script type="text/javascript">
//Fade in/out effect for cover images using jquery
$(document).ready(function() {
coverpics = $('.fbcovers img');
$(coverpics).fadeTo("fast", 0.70);
coverpics.mouseenter(OnEnter).mouseleave(OnLeave);
function OnEnter(){$(this).fadeTo("fast", 1);}
function OnLeave(){$(this).fadeTo("fast", 0.70);}
});
</script>
<link href="style.css" rel="stylesheet" type="text/css" />
</head>
<body>
<div align="center" class="fbpicwrapper">
<h1>Change Facebook Cover or Profile Pic with PHP</h1>
<div class="fbpic_desc">Please click on cover image you like the most for your profile!</div>
<ul class="fbcovers">
<li><a href="process.php?pid=1"><img src="cover_pics/cover1.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=2"><img src="cover_pics/cover2.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=3"><img src="cover_pics/cover3.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=4"><img src="cover_pics/cover4.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=5"><img src="cover_pics/cover5.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=6"><img src="cover_pics/cover6.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=7"><img src="cover_pics/cover7.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=8"><img src="cover_pics/cover8.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=9"><img src="cover_pics/cover9.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=10"><img src="cover_pics/cover10.jpg" width="850" height="315" border="0" /></a></li>
<li><a href="process.php?pid=11"><img src="cover_pics/cover11.jpg" width="850" height="315" border="0" /></a></li>
</ul>
</div>
</body>
</html>
Uploading image to Facebook
This is main page which does the task of posting cover picture on user's Facebook account. Once the image is uploaded, picture can be set as cover or profile picture.Permissions: To post pictures to user's wall, your application requires publish_action permission, and to access user photos user_photos permission is required. Without these Facebook permissions, your application can not post or access user photos. So we make sure our application has appropriate Facebook permissions before trying to change user cover or profile picture.PHP
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
<?php
session_start();
require_once('Facebook/autoload.php' );//include facebook api library
######### edit details ##########
$appId = 'xxxxx'; //Facebook App ID
$appSecret = 'xxxxxxxxxxxxxx'; // Facebook App Secret
$return_url = 'http://path-to-this-file/process.php'; //return url (url to script)
$homeurl = 'http://path-to-image-list/'; //return to home
$fbPermissions = 'publish_actions, user_photos'; //Required facebook permissions
##################################
if(isset($_GET["pid"])){
$_SESSION["pic_id"] = $_GET["pid"]; // Picture ID from Index page
}
switch($_SESSION["pic_id"])
{
case 1:
$PicLocation = 'cover_pics/cover1.jpg';
break;
case 2:
$PicLocation = 'cover_pics/cover2.jpg';
break;
case 3:
$PicLocation = 'cover_pics/cover3.jpg';
break;
case 4:
$PicLocation = 'cover_pics/cover4.jpg';
break;
case 5:
$PicLocation = 'cover_pics/cover5.jpg';
break;
case 6:
$PicLocation = 'cover_pics/cover6.jpg';
break;
case 7:
$PicLocation = 'cover_pics/cover7.jpg';
break;
case 8:
$PicLocation = 'cover_pics/cover8.jpg';
break;
case 9:
$PicLocation = 'cover_pics/cover9.jpg';
break;
case 10:
$PicLocation = 'cover_pics/cover10.jpg';
break;
case 11:
$PicLocation = 'cover_pics/cover11.jpg';
break;
default:
// header('Location: ' . $homeurl);
break;
}
$fb = new Facebook\Facebook([
'app_id' => $appId,
'app_secret' => $appSecret,
'default_graph_version' => 'v2.4'
]);
//try to get access token
try{
$helper = $fb->getRedirectLoginHelper();
$session = $helper->getAccessToken();
}catch(FacebookRequestException $ex){
die(" Facebook Message: " . $ex->getMessage());
} catch(Exception $ex){
die( " Message: " . $ex->getMessage());
}
//get picture ready for upload
$data = ['message' => '','source' => $fb->fileToUpload($PicLocation)];
//try upload photo to facebook wall
if($session){
try{
$photo_response = $fb->post('/me/photos', $data, $session);
$graph_node = $photo_response->getGraphNode();
} catch(FacebookRequestException $ex){
die(" Facebook Message: " . $ex->getMessage());
} catch(Exception $ex){
die( " Message: " . $ex->getMessage());
}
}else{
//if login requires redirect user to facebook login page
$login_url = $helper->getLoginUrl($return_url, array('scope'=> $fbPermissions));
header('Location: '. $login_url);
exit();
}
if(isset($graph_node["id"]) && is_numeric($graph_node["id"]))
{
/*
image is posted in user facebook account, but still we need to send user to facebook
so s/he can set cover or profile picture!
*/
//Get url of the picture just uploaded in user facebook account
$jsonurl = "https://graph.facebook.com/".$graph_node["id"]."?access_token=".$session;
$json = file_get_contents($jsonurl,0,null,null);
$json_output = json_decode($json);
/*
We can not set facebook cover or profile picture automatically,
So the trick is to post picture into user facebook account first
and then redirect them to a facebook profile page where they just have to click a button to set it.
*/
echo '<html><head><title>Update Image</title>';
echo '<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />';
echo '<link href="style.css" rel="stylesheet" type="text/css" />';
echo '</head><body>';
echo '<div align="center" class="fbpicwrapper">';
echo '<h1>Image is sent to your facebook account!</h1>';
echo '<div class="fbpic_desc">Click on desired button you want to do with this image!</div>';
echo '<div class="option_img"><img src="'.$json_output->source.'" /></div>';
/*
Links (buttons) below will send user to facebook page,
where they just need to crop or correct propertion of image and hit apply button.
*/
echo '<a class="button" target="_blank" href="http://www.facebook.com/profile.php?preview_cover='.$graph_node["id"].'">Make Your Profile Cover</a>';
echo '<a class="button" target="_blank" href="http://www.facebook.com/photo.php?fbid='.$graph_node["id"].'&type=1&makeprofile=1&makeuserprofile=1">Make Your Profile Picture</a>';
echo '<a class="button" href="'.$homeurl.'">Back to main Page.</a>';
echo '</div>';
echo '</body></html>';
}
?>