Select Box Change Dependent Options dynamically (JavaScript Object)
There are different solutions you can find that shows how to change/load the content in child <SELECT> element, depending on the selection of the parent <SELECT> options. Most examples uses Ajax or page refresh methods to achieve, which of-course are useful depending on the circumstance. But if you wish to use another simple method using pure JavaScript, here’s how you can do it.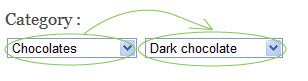
HTML
You can start by inserting two HTML <SELECT> elements in your page. Which could be identified with their ID names, parent and child.1234
<select id="parent_select">
<option>----</option>
</select>
<select id="child_select"></select>
Create JavaScript Objects
First create a list of objects and its properties like shown in the example below. I have created a list of random stuff below, in similar way you can create a list as per your requirements.JS
123456
var mList = {
Chocolates : ['Bournville', 'Snickers', 'Twix', 'Ferrero Rocher', 'Dark Chocolate', 'Milk chocolate', 'White chocolate', 'Gianduja chocolate'],
Vegetables : ['Broccoli', 'Cabbage', 'Carrot', 'Cauliflower'],
Icecreams : ['Black Raspberry', 'Frozen pudding', 'Neapolitan', 'Strawberry', 'Vanilla', 'Chokecherry', 'Frozen yogurt', 'Booza', 'Frozen yogurt', 'Ice milk'],
Mobiles : ['Apple iPhone', 'Samsung Galaxy', 'Realme', 'Huawei', 'OnePlus', 'Asus ROG', 'Xiaomi', 'Vivo']
};
On Parent Change
Once we have the list of Objects, we can automatically populated the parent SELECT box with KEY names as options, and then load its child objects dynamically, when user selects the parent key in the SELECT box.JS
1234567891011121314151617
el_parent = document.getElementById("parent_select"); //parent select element
el_child = document.getElementById("child_select"); //child select element
for (key in mList) { //populate the parent select element with array key
el_parent.innerHTML = el_parent.innerHTML + '<option>'+ key +'</option>';
}
//add event listener to parent select, so that when user selects an option, child gets populated
el_parent.addEventListener('change', function populate_child(e){
el_child.innerHTML = '';
itm = e.target.value;
if(itm in mList){
for (i = 0; i < mList[itm].length; i++) {
el_child.innerHTML = el_child.innerHTML + '<option>'+ mList[itm][i] +'</option>';
}
}
});
JQUERY
12345678910111213141516171819202122232425262728293031
<div class="wrapper">
<select id="parent_select"><option>----</option></select>
<select id="child_select"></select>
</div>
<script language="javascript" type="text/javascript">
var mList = {
Chocolates : ['Bournville', 'Snickers', 'Twix', 'Ferrero Rocher', 'Dark Chocolate', 'Milk chocolate', 'White chocolate', 'Gianduja chocolate'],
Vegetables : ['Broccoli', 'Cabbage', 'Carrot', 'Cauliflower'],
Icecreams : ['Black Raspberry', 'Frozen pudding', 'Neapolitan', 'Strawberry', 'Vanilla', 'Chokecherry', 'Frozen yogurt', 'Booza', 'Frozen yogurt', 'Ice milk'],
Mobiles : ['Apple iPhone', 'Samsung Galaxy', 'Realme', 'Huawei', 'OnePlus', 'Asus ROG', 'Xiaomi', 'Vivo']
};
el_parent = document.getElementById("parent_select");
el_child = document.getElementById("child_select");
for (key in mList) {
el_parent.innerHTML = el_parent.innerHTML + '<option>'+ key +'</option>';
}
el_parent.addEventListener('change', function populate_child(e){
el_child.innerHTML = '';
itm = e.target.value;
if(itm in mList){
for (i = 0; i < mList[itm].length; i++) {
el_child.innerHTML = el_child.innerHTML + '<option>'+ mList[itm][i] +'</option>';
}
}
});
</script>
Demo
Category :