PHP mail with Multiple Attachments
In previous example we’ve learned how to send PHP mail with an attachment, and I have also created separate Ajax tutorial for the same. But some of you also want to send multiple attachments, so today I am going to refine the PHP code to show you how we can send multiple attachments with PHP mail.Markup
First we need to create HTML multipart/form-data form with file input fields.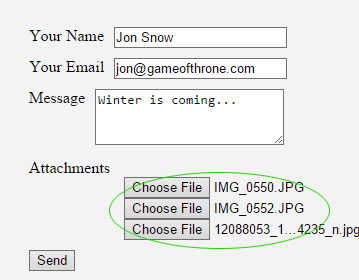
HTML
1234567891011121314151617181920212223242526
<form method="post" action="send_attachments.php" enctype="multipart/form-data">
<label>
<span>Your Name</span>
<input type="text" value="Jon Snow" name="s_name" />
</label>
<label>
<span>Your Email</span>
<input type="email" value="" name="s_email" />
</label>
<label>
<span>Message</span>
<textarea name="s_message"></textarea>
</label>
<label>
<span>Attachments</span>
<!-- File input fields, you can add as many as required-->
<input type="file" name="file[]" />
<input type="file" name="file[]" />
<input type="file" name="file[]" />
<!-- OR -->
<! -- <input type="file" name="file[]" multiple="multiple" /> -->
</label>
<label>
<input type="submit" value="Send" />
</label>
</form>
PHP Mail
Once you are done with HTML part, you can examine the code below and copy it in a file and name it as send_attachments.php, which is the PHP file pointed in action parameter in HTML form above.PHP
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778798081828384858687888990
<?php
if($_POST && isset($_FILES['file']))
{
$recipient_email = "[email protected]"; //recepient
$from_email = "info@your_domain.com"; //from email using site domain.
$subject = "Attachment email from your website!"; //email subject line
$sender_name = filter_var($_POST["s_name"], FILTER_SANITIZE_STRING); //capture sender name
$sender_email = filter_var($_POST["s_email"], FILTER_SANITIZE_STRING); //capture sender email
$sender_message = filter_var($_POST["s_message"], FILTER_SANITIZE_STRING); //capture message
$attachments = $_FILES['file'];
//php validation
if(strlen($sender_name)<4){
die('Name is too short or empty');
}
if (!filter_var($sender_email, FILTER_VALIDATE_EMAIL)) {
die('Invalid email');
}
if(strlen($sender_message)<4){
die('Too short message! Please enter something');
}
$file_count = count($attachments['name']); //count total files attached
$boundary = md5("sanwebe.com");
if($file_count > 0){ //if attachment exists
//header
$headers = "MIME-Version: 1.0\r\n";
$headers .= "From:".$from_email."\r\n";
$headers .= "Reply-To: ".$sender_email."" . "\r\n";
$headers .= "Content-Type: multipart/mixed; boundary = $boundary\r\n\r\n";
//message text
$body = "--$boundary\r\n";
$body .= "Content-Type: text/plain; charset=ISO-8859-1\r\n";
$body .= "Content-Transfer-Encoding: base64\r\n\r\n";
$body .= chunk_split(base64_encode($sender_message));
//attachments
for ($x = 0; $x < $file_count; $x++){
if(!empty($attachments['name'][$x])){
if($attachments['error'][$x]>0) //exit script and output error if we encounter any
{
$mymsg = array(
1=>"The uploaded file exceeds the upload_max_filesize directive in php.ini",
2=>"The uploaded file exceeds the MAX_FILE_SIZE directive that was specified in the HTML form",
3=>"The uploaded file was only partially uploaded",
4=>"No file was uploaded",
6=>"Missing a temporary folder" );
die($mymsg[$attachments['error'][$x]]);
}
//get file info
$file_name = $attachments['name'][$x];
$file_size = $attachments['size'][$x];
$file_type = $attachments['type'][$x];
//read file
$handle = fopen($attachments['tmp_name'][$x], "r");
$content = fread($handle, $file_size);
fclose($handle);
$encoded_content = chunk_split(base64_encode($content)); //split into smaller chunks (RFC 2045)
$body .= "--$boundary\r\n";
$body .="Content-Type: $file_type; name=\"$file_name\"\r\n";
$body .="Content-Disposition: attachment; filename=\"$file_name\"\r\n";
$body .="Content-Transfer-Encoding: base64\r\n";
$body .="X-Attachment-Id: ".rand(1000,99999)."\r\n\r\n";
$body .= $encoded_content;
}
}
}else{ //send plain email otherwise
$headers = "From:".$from_email."\r\n".
"Reply-To: ".$sender_email. "\n" .
"X-Mailer: PHP/" . phpversion();
$body = $sender_message;
}
$sentMail = @mail($recipient_email, $subject, $body, $headers);
if($sentMail) //output success or failure messages
{
die('Thank you for your email');
}else{
die('Could not send mail! Please check your PHP mail configuration.');
}
}
?>
Check-out Ajax Contact Form with an Attachment for Ajax tutorial.