Creating Simple Hover Fade jQuery Plugin
Since the launch of jQuery in January 2006, jQuery has quickly become the most popular and JavaScript library of choice for all web developers. There are hundreds of plugins available for almost anything you may want to do with jQuery, you can just download and start using those wonderful plugins in your project right away. But there are times, when you want to learn to create your own jQuery plugin, let's find out how hard/easy is it to create a jQuery plugin.In this tutorial we will look at very basics of jQuery Plugin creation, we will step by step create a plugin named hoverfade. This plugin will have just one task to accomplish, fade-in and fade-out the element when the mouse pointer enters and leaves its area. Plugin works pretty much like CSS hover effect and it also looks cool.Loading the Library
Let us first load jQuery library, as we all know javascript should loaded within the head section of the document body, and right after that we will load our plugin file hoverfade.jquery.js.JQUERY
- 1
- 2
- 3
- 4
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.js"></script>
<script src="hoverfade.jquery.js"></script>
</head>
Inside Plugin File "hoverfade.jquery.js"
If you open the plugin file and take a look inside, you'll see that I have added "hoverfade" function to $.fn, it makes our plugin act like any other jQuery object method, we can now simply load our plugin file and call this function in the HTML page. But wait, plugin is not complete yet, we still need to program it to do the task we want.In next line, $(this) represents a HTML element, Once we select the element with $ function, we will have all the jQuery methods we need, we can call methods like .click() .hide() etc.In our case we want to set initial opacity of the element to 30%, so we just use .fadeTo() method to fade the element. Next I've created two functions OnEnter() and OnLeave(), which I've called these functions within mouseenter() and mouseleave() jQuery method.JQUERY
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
(function($) {
$.fn.hoverfade = function() //wrap our plugin codes in jquery function property.
{
$(this).fadeTo("fast", 0.30); // on load, reduce element(this) opacity to 30%
this.mouseenter(OnEnter).mouseleave(OnLeave); //On mouseenter / mouseleave, call our functions
function OnEnter() //Executes on mouseenter
{
$(this).fadeTo("fast", 1); //Bring opacity back to 100%
}
function OnLeave() //Executes on mouseleave
{
$(this).fadeTo("fast", 0.30); //recude opacity to 30%
}
}
})(jQuery);
Writing jQuery Plugin
You see, the whole code inside the plugin is very simple, and self explanatory. On page load, opacity of the elements is reduced to 30%, when user hovers the mouse on the element opacity is increased to 100%, and again on mouse leave opacity is reduced back to 30%.Normally without the plugin, the jQuery code that fades the element looks like below, we simply take the idea and convert it to jQuery plugin.JQUERY
- 1
- 2
- 3
- 4
- 5
<script>
$('.fader').mouseenter(function() {
$('.fader').fadeTo("fast", 0.3);
});
</script>
JQUERY
- 1
- 2
- 3
- 4
- 5
- 6
(function($) {
$.fn.XXXX = function()
{
//Plugin stuff
}
})(jQuery);
Applying the Plugin
You can now apply the plugin to the elements. jQuery code below will do exactly that:JQUERY
- 1
- 2
- 3
- 4
- 5
<script type="text/javascript">
$(document).ready(function() {
$('.fader').hoverfade();
});
</script>
HTML File
The complete HTML file, with jQuery library and our plugin loaded. Noticed the plugin is applied to all the div element which has class "fader", it contains images inside them, just hover over the element to see it in action. Similarly you can apply this plugin to other elements as well.JQUERY
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
<html>
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.6.2/jquery.js"></script>
<script src="hoverfade.jquery.js"></script>
<script type="text/javascript">
$(document).ready(function() {
$('.fader').hoverfade();
});
</script>
<style>
.fader{float:left;margin:10px;}
</style>
</head>
<body>
<div class="fader"><img src="charlize-throne.jpg" id="image" width="100" height="100"></div>
<div class="fader"><img src="charlize-throne2.jpg" id="image" width="100" height="100"></div>
<div class="fader"><img src="charlize-throne3.jpg" id="image" width="100" height="100"></div>
</body>
</html>
Demo
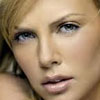

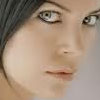