Creating Simple Sliding Menu Quickly (CSS & jQuery)
There are plenty of Responsive menus on the net, which you can incorporate within your project to make an exciting navigation system. But more often than not we end up fixing and tweaking things with those menus! So today let's focus on creating our own simple sliding navigation menu which will fit any screen size and also looks great.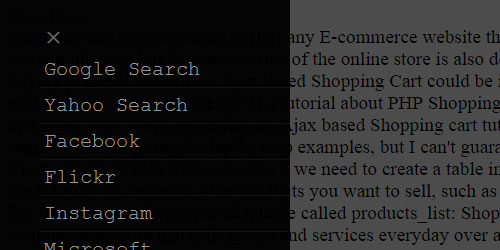
HTML
Let's start by writing the HTML code for the menu. As you can see we have one overly element, a close button and rest are menu links.HTML
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
<div class="menu-overlay"></div>
<a href="#" class="menu-open">Open Menu</a>
<div class="side-menu-wrapper">
<a href="#" class="menu-close">×</a>
<ul>
<li><a href="http://www.google.com" target="_blank" rel="nofollow">Google Search</a></li>
<li><a href="http://www.yahoo.com" target="_blank" rel="nofollow">Yahoo Search</a></li>
<li><a href="http://www.facebook.com" target="_blank" rel="nofollow">Facebook</a></li>
<li><a href="http://www.flickr.com" target="_blank" rel="nofollow">Flickr</a></li>
</ul>
</div>
CSS Style
I have applied the CSS transition property, which will make our menu sliding smooth. To position the menu bar to right or left of the browser, just change left to right. You must also need to make changes in jQuery code below.CSS
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
.side-menu-wrapper { /* style menu wrapper */
background: rgba(0,0,0,.95);
padding: 40px 0 0 40px;
position: fixed; /* Fixed position */
top: 0;
right: 0; /* Sidebar initial position. "right" for right positioned menu */
height: 100%;
z-index: 2;
transition: 0.5s; /* CSS transition speed */
width: 250px;
font: 20px "Courier New", Courier, monospace;
box-sizing: border-box;
}
.side-menu-wrapper > ul{ /* css ul list style */
list-style:none;
padding:0;
margin:0;
overflow-y: auto; /* enable scroll for menu items */
height:95%;
}
.side-menu-wrapper > ul > li > a { /* links */
display: block;
border-bottom: 1px solid #131313;
padding: 6px 4px 6px 4px;
color: #989898;
transition: 0.3s;
text-decoration: none;
}
.side-menu-wrapper > a.menu-close { /* close button */
padding: 8px 0 4px 23px;
color: #6B6B6B;
display: block;
margin: -30px 0 -10px -20px;
font-size: 35px;
text-decoration: none;
}
.menu-overlay { /* overlay */
height: 100%;
width: 0;
position: fixed;
z-index: 1;
top: 0;
left: 0;
background-color: rgba(0,0,0,.7);
overflow-y: auto;
overflow-x: hidden;
text-align: center;
opacity: 0;
transition: opacity 1s;
}
jQuery
In jQuery code we simply change the CSS property of the menu using jQuery .CSS(), since we've enabled CSS transition, it should take care of the sliding animation without the help of jQuery.JQUERY
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
var slide_wrp = ".side-menu-wrapper"; //Menu Wrapper
var open_button = ".menu-open"; //Menu Open Button
var close_button = ".menu-close"; //Menu Close Button
var overlay = ".menu-overlay"; //Overlay
//Initial menu position
$(slide_wrp).hide().css( {"right": -$(slide_wrp).outerWidth()+'px'}).delay(50).queue(function(){$(slide_wrp).show()});
$(open_button).click(function(e){ //On menu open button click
e.preventDefault();
$(slide_wrp).css( {"right": "0px"}); //move menu right position to 0
setTimeout(function(){
$(slide_wrp).addClass('active'); //add active class
},50);
$(overlay).css({"opacity":"1", "width":"100%"});
});
$(close_button).click(function(e){ //on menu close button click
e.preventDefault();
$(slide_wrp).css( {"right": -$(slide_wrp).outerWidth()+'px'}); //hide menu by setting right position
setTimeout(function(){
$(slide_wrp).removeClass('active'); // remove active class
},50);
$(overlay).css({"opacity":"0", "width":"0"});
});
$(document).on('click', function(e) { //Hide menu when clicked outside menu area
if (!e.target.closest(slide_wrp) && $(slide_wrp).hasClass("active")){ // check menu condition
$(slide_wrp).css( {"right": -$(slide_wrp).outerWidth()+'px'}).removeClass('active');
$(overlay).css({"opacity":"0", "width":"0"});
}
});