Generate Facebook ID Card with PHP
You may have came across some some horoscope or fun images of your friends, aren't they just fun? well, why not create some fun page like that on your website to allow your visitors to generate a Facebook ID card, which they can save on their computer or publish on Facebook wall. Let's focus on creating some authentic looking "Facebook ID" for the users using PHP and PHP Facebook SDK.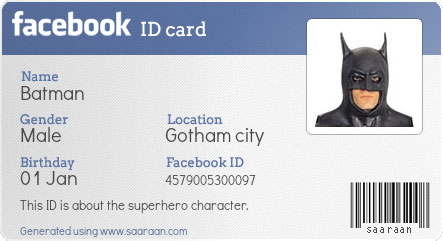
Facebook SDK
We start by including Facebook PHP SDK file in our PHP file, and then writing basic code blocks, which will connect to Facebook and fetch appropriate user data for us to continue further. Everything takes place in just one PHP file here, here's a look at our initial PHP code for Facebook SDK:PHP
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
//include facebook SDK
include_once("inc/facebook.php");
//New Facebook object with your app id and app secret
$facebook = new Facebook(array(
'appId' => $appId,
'secret' => $appSecret,
));
//get facebook user
$fbuser = $facebook->getUser();
if(!$fbuser) //if user is null
{
//show login button
}
else
{
//do stuff like merging image and
}
Generating ID card
Once user returns back to page after the authentication, we copy user profile image to local server using PHP copy() function, and then we need to merge profile image with blank ID card image template, and write user name, birthday, hometown to the image using TrueType fonts.PHP
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
//copy facebook image to local folder
copy('http://graph.facebook.com/USER_ID/picture?width=100&height=100','Local_folder/USER_ID.jpg');
//merge two images (profile picture on id card image template)
imagecopymerge($dest, $src, 320, 32, 0, 0, 100, 100, 100);
//write on merged image using a font
imagettftext($dest, 10, 0, 170, 190, 'color' , 'DidactGothic.ttf', 'Text to write');
Finishing
Below you'll find entire PHP code that generates Facebook ID card. The comment lines should make things easy for you to understand, you can also download the sample files and create your own version of ID card.PHP
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
- 44
- 45
- 46
- 47
- 48
- 49
- 50
- 51
- 52
- 53
- 54
- 55
- 56
- 57
- 58
- 59
- 60
- 61
- 62
- 63
- 64
- 65
- 66
- 67
- 68
- 69
- 70
- 71
- 72
- 73
- 74
- 75
- 76
- 77
- 78
- 79
- 80
- 81
- 82
- 83
- 84
- 85
- 86
- 87
- 88
- 89
- 90
- 91
- 92
- 93
- 94
- 95
- 96
- 97
- 98
- 99
- 100
- 101
- 102
- 103
- 104
- 105
- 106
- 107
- 108
- 109
- 110
- 111
- 112
- 113
- 114
- 115
- 116
- 117
- 118
- 119
- 120
- 121
- 122
- 123
- 124
- 125
- 126
- 127
- 128
- 129
- 130
- 131
- 132
- 133
- 134
- 135
- 136
- 137
- 138
- 139
- 140
- 141
######### edit details ##########
$appId = 'xxxxxxxxxxx'; //Facebook App ID
$appSecret = 'xxxxxxxxxxxxxxxxxxxxxxxx'; // Facebook App Secret
$return_url = 'http://www.sitename.com/get_fb_id/'; //return url (url to script)
$temp_folder = 'tmp/'; //temp dir path to store images
$fbPermissions = 'publish_stream,user_hometown,user_birthday'; //Required facebook permissions
$image_id_png = 'assets/id.png'; // id card image template path
$font = 'assets/fonts/DidactGothic.ttf'; //font used
#################################
// make sure curl is enabled and working
if (!in_array ('curl', get_loaded_extensions())){die('curl required!');}
//include facebook SDK
include_once("inc/facebook.php");
//Facebook API
$facebook = new Facebook(array(
'appId' => $appId,
'secret' => $appSecret,
));
if(isset($_GET["logout"]) && $_GET["logout"]==1)
{
//Destroy the current session and logout user
$facebook->destroySession();
header('Location: '.$return_url);
}
//get currently logged-in Facebook user details
$fbuser = $facebook->getUser();
if(!$fbuser)
{
//new users get to see this login button
$loginUrl = $facebook->getLoginUrl(array('scope' => $fbPermissions,'redirect_uri'=>$return_url));
echo '<div style="margin:20px;text-align:center;"><a href="'.$loginUrl.'"><img src="assets/facebook-login.png" /></a></div>';
}
else
{
//get user profile
try {
$user_profile = $facebook->api('/me');
//list of user granted permissions
$user_permissions = $facebook->api("/me/permissions");
} catch (FacebookApiException $e) {
echo $e;
$fbuser = null;
}
//login url
$loginUrl = $facebook->getLoginUrl(array('scope' => $fbPermissions,'redirect_uri'=>$return_url));
// permission required to proceed
$permissions_needed = explode(',',$fbPermissions);
//loop thrugh each permission
foreach($permissions_needed as $per)
{
//if more permission needed show login link
if (!array_key_exists($per, $user_permissions['data'][0])) {
die('<div>We need additional '.$per.' permission to continue, <a href="'.$loginUrl.'">click here</a>!</div>');
}
}
//display logout url
echo '<div>'.$user_profile["name"].' [<a href="?logout=1">Log Out</a>]</div>';
###### start generating ID ##########
//copy user profile image from facebook in temp folder
if(!copy('http://graph.facebook.com/'.$fbuser.'/picture?width=100&height=100',$temp_folder.$fbuser.'.jpg'))
{
die('Could not copy image!');
}
##### start generating Facebook ID ########
$dest = imagecreatefrompng($image_id_png); // source id card image template
$src = imagecreatefromjpeg($temp_folder.$fbuser.'.jpg'); //facebook user image stored in our temp folder
imagealphablending($dest, false);
imagesavealpha($dest, true);
//merge user picture with id card image template
//need to play with numbers here to get alignment right
imagecopymerge($dest, $src, 320, 32, 0, 0, 100, 100, 100);
//colors we use for font
$facebook_blue = imagecolorallocate($dest, 81, 103, 147); // Create blue color
$facebook_grey = imagecolorallocate($dest, 74, 74, 74); // Create grey color
//Texts to embed into id card image template
$txt_user_id = $fbuser;
$txt_user_name = isset($user_profile['name'])?$user_profile['name']:'No Name';
$txt_user_gender = isset($user_profile['gender'])?$user_profile['gender']:'No gender';
$txt_user_hometown = isset($user_profile['hometown'])?$user_profile['hometown']['name']:'Unknown';
$txt_user_birth = isset($user_profile['birthday'])?$user_profile['birthday']:'00/00/0000';
$user_text = 'Your source for Google+ and hangout graphics for free.';
$txt_credit = 'Generated using www.saaraan.com';
//format birthday, not showing whole birth date!
$fb_birthdate = date($txt_user_birth);
$sort_birthdate = strtotime($fb_birthdate);
$for_birthdate = date('d M', $sort_birthdate);
imagealphablending($dest, true); //bring back alpha blending for transperent font
imagettftext($dest, 10, 0, 170, 190, $facebook_grey , $font, $txt_user_id); //Write user id to id card
imagettftext($dest, 15, 0, 25, 105, $facebook_grey, $font, $txt_user_name); //Write name to id card
imagettftext($dest, 15, 0, 25, 147, $facebook_grey, $font, $txt_user_gender); //Write gender to id card
imagettftext($dest, 15, 0, 170, 147, $facebook_grey, $font, $txt_user_hometown); //Write hometown to id card
imagettftext($dest, 15, 0, 25, 190, $facebook_grey, $font, $for_birthdate); //Write birthday to id card
imagettftext($dest, 10, 0, 25, 215, $facebook_grey, $font, $user_text); //Write custom text to id card
imagettftext($dest, 8, 0, 25, 240, $facebook_blue, $font, $txt_credit); //Write credit link to id card
imagepng($dest, $temp_folder.'id_'.$fbuser.'.jpg'); //save id card in temp folder
//now we have generated ID card, we can display it on browser or post it on facebook
echo '<img src="tmp/id_'.$fbuser.'.jpg" >'; //display saved id card
/* or output image to browser directly
header('Content-Type: image/png');
imagepng($dest);
*/
/* //Post ID card to User Wall
$post_url = '/'.$fbuser.'/photos';
//posts message on page statues
$msg_body = array(
'source'=>'@'.'tmp/id_'.$fbuser.'.jpg',
'message' => 'interesting ID';
);
$postResult = $facebook->api($post_url, 'post', $msg_body );
*/
imagedestroy($dest);
imagedestroy($src);
}