Extract URL content like Facebook with PHP and jQuery
You may have seen Facebook's technique that extracts content of remote URL when a user types a URL in the status text-box filed. In this article, we will try to create a similar type of Ajax based URL content extractor using jQuery and PHP.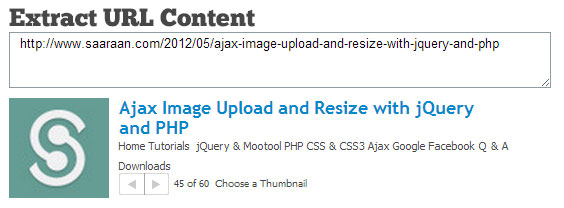
Index Page
Let's start with jQuery code, when user types the URL in the text box, we will extract the first typed URL using regular expression, and then we make Ajax request to PHP file, which will extract the content from URL and send content back to index page as JSON format, which we will output in an element using jQuery.JQUERY
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
$(document).ready(function() {
var getUrl = $('#get_url'); //url to extract from text field
getUrl.keyup(function() { //user types url in text field
//url to match in the text field
var match_url = /\b(https?):\/\/([\-A-Z0-9.]+)(\/[\-A-Z0-9+&@#\/%=~_|!:,.;]*)?(\?[A-Z0-9+&@#\/%=~_|!:,.;]*)?/i;
//continue if matched url is found in text field
if (match_url.test(getUrl.val())) {
$("#results").hide();
$("#loading_indicator").show(); //show loading indicator image
var extracted_url = getUrl.val().match(match_url)[0]; //extracted first url from text filed
//ajax request to be sent to extract-process.php
$.post('extract-process.php',{'url': extracted_url}, function(data){
extracted_images = data.images;
total_images = parseInt(data.images.length-1);
img_arr_pos = total_images;
if(total_images>0){
inc_image = '<div class="extracted_thumb" id="extracted_thumb"><img src="'+data.images[img_arr_pos]+'" width="100" height="100"></div>';
}else{
inc_image ='';
}
//content to be loaded in #results element
var content = '<div class="extracted_url">'+ inc_image +'<div class="extracted_content"><h4><a href="'+extracted_url+'" target="_blank">'+data.title+'</a></h4><p>'+data.content+'</p><div class="thumb_sel"><span class="prev_thumb" id="thumb_prev"> </span><span class="next_thumb" id="thumb_next"> </span> </div><span class="small_text" id="total_imgs">'+img_arr_pos+' of '+total_images+'</span><span class="small_text"> Choose a Thumbnail</span></div></div>';
//load results in the element
$("#results").html(content); //append received data into the element
$("#results").slideDown(); //show results with slide down effect
$("#loading_indicator").hide(); //hide loading indicator image
},'json');
}
});
});
Thumbnail Selection
In PHP page we will output title, page content and image URLs for thumbnail in JSON format. We can make a neat thumbnail navigation for the users, which will allow them to select an image for the thumbnail.JQUERY
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
$(document).ready(function() {
//user clicks previous thumbail
$("body").on("click","#thumb_prev", function(e){
if(img_arr_pos>0)
{
img_arr_pos--; //thmubnail array position decrement
//replace with new thumbnail
$("#extracted_thumb").html('<img src="'+extracted_images[img_arr_pos]+'" width="100" height="100">');
//replace thmubnail position text
$("#total_imgs").html((img_arr_pos) +' of '+ total_images);
}
});
//user clicks next thumbail
$("body").on("click","#thumb_next", function(e){
if(img_arr_pos<total_images)
{
img_arr_pos++; //thmubnail array position increment
//replace with new thumbnail
$("#extracted_thumb").html('<img src="'+extracted_images[img_arr_pos]+'" width="100" height="100">');
//replace thmubnail position text
$("#total_imgs").html((img_arr_pos) +' of '+ total_images);
}
});
});
Mark Up
Here's how HTML, the user typing goes in textarea field, and the results will be loaded in Div element with id called results. I have tried to make it look like Facebook status box, but it may require bit more CSS to get it right.HTML
- 1
- 2
- 3
- 4
- 5
- 6
<div class="extract_url">
<img id="loading_indicator" src="images/ajax-loader.gif">
<textarea id="get_url" placeholder="Enter Your URL here" class="get_url_input" spellcheck="false" ></textarea>
<div id="results">
</div>
</div>
PHP
In our PHP page, we will be including PHP Simple HTML DOM Parser, which lets us easily handle and get content in HTML elements. Please note that PHP Simple HTML DOM Parser requires PHP 5+. The code below extracts the remote content title, body plain text and images for the thumbnails, and outputs them in JSON format.PHP
- 1
- 2
- 3
- 4
- 5
- 6
- 7
- 8
- 9
- 10
- 11
- 12
- 13
- 14
- 15
- 16
- 17
- 18
- 19
- 20
- 21
- 22
- 23
- 24
- 25
- 26
- 27
- 28
- 29
- 30
- 31
- 32
- 33
- 34
- 35
- 36
- 37
- 38
- 39
- 40
- 41
- 42
- 43
<?php
if(isset($_POST["url"]))
{
$get_url = $_POST["url"];
//Include PHP HTML DOM parser (requires PHP 5 +)
include_once("include/simple_html_dom.inc.php");
//get URL content
$get_content = file_get_html($get_url);
//Get Page Title
foreach($get_content->find('title') as $element)
{
$page_title = $element->plaintext;
}
//Get Body Text
foreach($get_content->find('body') as $element)
{
$page_body = trim($element->plaintext);
$pos=strpos($page_body, ' ', 200); //Find the numeric position to substract
$page_body = substr($page_body,0,$pos ); //shorten text to 200 chars
}
$image_urls = array();
//get all images URLs in the content
foreach($get_content->find('img') as $element)
{
/* check image URL is valid and name isn't blank.gif/blank.png etc..
you can also use other methods to check if image really exist */
if(!preg_match('/blank.(.*)/i', $element->src) && filter_var($element->src, FILTER_VALIDATE_URL))
{
$image_urls[] = $element->src;
}
}
//prepare for JSON
$output = array('title'=>$page_title, 'images'=>$image_urls, 'content'=> $page_body);
echo json_encode($output); //output JSON data
}
?>