Creating Custom WordPress Widget on certain pages
Recently I needed to create a Facebook likebox WordPress widget for my friend, which of course can be easily created using a WordPress text widget, but since my friend is new to WordPress and has no clue about coding, and to make matters worse, she wanted to display it only on certain pages like single and category pages! So, I decided to quickly create a custom WordPress Widget, which should be flexible enough for her needs.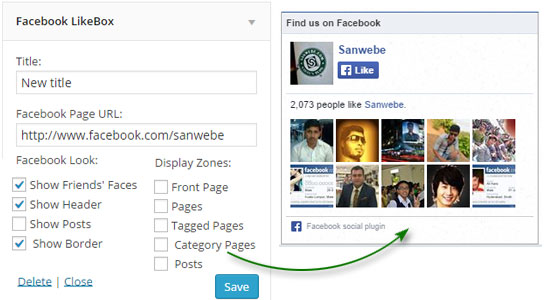
Getting Started
You can create your own separate Widget Plugin, which can be installed and activated in your admin plugin page, but as many people, I prefer to put my widget code in default theme’s functions.php file, which is located in your active theme folder i.e (/wp-content/themes/YOUR-THEME). This way our custom widget works perfectly and I don’t need to install additional plugin.Writing Basic Widget Code
Open your theme’s function.php (Create if you don’t find one), and copy/paste this PHP code anywhere within function.php and save the file. Now go back to your admin widget page, you should see “Facebook Likebox” widget in Available widget section. Just drag it to sidebar to see it in action.PHP
12345678910111213141516171819202122232425262728293031323334353637383940414243
//extend the standard WP_Widget class
class facebook_likebox_widget extends WP_Widget {
//construct widget names and descriptions
public function __construct() {
parent::__construct(
'facebook_likebox_widget', // Base ID
__('Facebook LikeBox', 'text_domain'), // Name
array( 'description' => __( 'Customized Facebook LikeBox Widget for your WordPress.', 'text_domain' ), ) // Args
);
}
//output widget content
public function widget( $args, $instance ) {
//facebook Likebox code
echo $args['before_widget'];
echo '<div class="fb-like-box" data-href="https://www.facebook.com/sanwebe" data-colorscheme="light" data-show-faces="true" data-header="true" data-stream="false" data-show-border="true"></div>';
echo '<div id="fb-root"></div>';
echo '<script>(function(d, s, id) {';
echo 'var js, fjs = d.getElementsByTagName(s)[0];';
echo 'if (d.getElementById(id)) return;';
echo 'js = d.createElement(s); js.id = id;';
echo 'js.src = "//connect.facebook.net/en_US/sdk.js#xfbml=1&appId=365936720119443&version=v2.0";';
echo 'fjs.parentNode.insertBefore(js, fjs);';
echo '}(document, \'script\', \'facebook-jssdk\'));</script>';
echo $args['after_widget'];
}
//option for admin
public function form( $instance ) {
//do stuff
}
// processes widget options to be saved
public function update( $new_instance, $old_instance ) {
//do stuff
}
}
//register widget using the widgets_init
add_action( 'widgets_init', 'reg_facebook_likebox_widget' );
function reg_facebook_likebox_widget() {
register_widget( 'facebook_likebox_widget' );
}
- Extend WP_Widget class as shown in code above.
- function __construct() : Define widget name, desc in your Widget Class.
- function widget() : HTML content output for your widget.
- function form() : HTML options form for user in admin section.
- function update() : This function saves options provided by user in option form.
- Register Widget using widgets_init.
Writing Customizable Widget
You can play with code above in your functions.php file, and when you think you are more comfortable, we can extend our Widget code further. Since our Facebook like widget will have many options, such as Facebook Page URL field, width, height fields and most importantly option to choose pages where user will be displaying the widget. I’ve made several changes in widget code adding HTML fields in function form(). I have also made changes in function update() and function widget() accordingly. You can see the changes in our complete Facebook Likebox widget code below:PHP
123456789101112131415161718192021222324252627282930313233343536373839404142434445464748495051525354555657585960616263646566676869707172737475767778798081828384858687888990919293949596979899100101102103104105106107108109110111112113114115116117118119120121122123124125126127128129130131132133134135136137138139140141142143144145146147148149150151152153154155156157158159160161162163164165166167
class facebook_likebox_widget extends WP_Widget{
public function __construct() {
parent::__construct(
'facebook_likebox_widget', // Base ID
__('Facebook LikeBox', 'text_domain'), // Name
array( 'description' => __( 'Customized Facebook LikeBox Widget for your WordPress.', 'text_domain' ), ) // Args
);
}
//output widget content
public function widget( $args, $instance ) {
//Display widget according to user settings
$display_widget = false;
if(is_front_page() && $instance['ifront_page']){
$display_widget = true;
}
if(is_page() && $instance['ipages']){
$display_widget = true;
}
if(is_tag() && $instance['itags']){
$display_widget = true;
}
if(is_category() && $instance['icats']){
$display_widget = true;
}
if(is_single() && $instance['iposts']){
$display_widget = true;
}
if($display_widget){
//output widget
echo $args['before_widget'];
if ( ! empty( $instance['title'] ) ) {
echo $args['before_title'] . apply_filters( 'widget_title', $instance['title'] ). $args['after_title']; //title
}
$facebook_page_url = (!empty($instance['fb_page_url'])) ? $instance['fb_page_url'] : ''; //fb page url
$fb_likebox_color = (!empty($instance['fb_likebox_color'])) ? 'data-colorscheme="'.$instance['fb_likebox_color'].'"' : 'data-colorscheme="light"'; //fb color scheme
$ishow_face = ($instance['ishow_face']) ? 'data-show-faces="true"' : 'data-show-faces="false"'; //fb show faces
$ishow_header = ($instance['ishow_header']) ? 'data-header="true"' : 'data-header="false"'; //fb show header
$ishow_posts = ($instance['ishow_posts']) ? 'data-stream="true"' : 'data-stream="false"'; //fb show stream posts
$ishow_border = ($instance['ishow_border']) ? 'data-show-border="true"' : 'data-show-border="false"'; //fb show border
//facebook Likebox code
echo '<div class="fb-like-box" data-href="'.$facebook_page_url.'" '.$fb_likebox_color.' '.$ishow_face.' '.$ishow_header.' '.$ishow_posts.' '.$ishow_border.'></div>';
echo '<div id="fb-root"></div>';
echo '<script>(function(d, s, id) {';
echo 'var js, fjs = d.getElementsByTagName(s)[0];';
echo 'if (d.getElementById(id)) return;';
echo 'js = d.createElement(s); js.id = id;';
echo 'js.src = "//connect.facebook.net/en_US/sdk.js#xfbml=1&appId=365936720119443&version=v2.0";';
echo 'fjs.parentNode.insertBefore(js, fjs);';
echo '}(document, \'script\', \'facebook-jssdk\'));</script>';
echo $args['after_widget'];
}
}
//display options for widget admin
public function form( $instance ){
//title of widget
$title = (isset($instance[ 'title' ]))? $instance[ 'title' ] : __( 'New title', 'text_domain' );
echo '<p>';
echo '<label for="' .$this->get_field_id( 'title' ). '">' ._e( 'Title:' ). '</label> ';
echo '<input class="widefat" id="'. $this->get_field_id( 'title' ) .'" name="'. $this->get_field_name( 'title' ) .'" type="text" value="'. esc_attr( $title ) .'">';
echo '</p>';
//Facebook Page URL
$fb_page_url = (isset($instance[ 'fb_page_url' ]))? $instance[ 'fb_page_url' ] : __( 'http://www.facebook.com/sanwebe', 'text_domain' );
echo '<p>';
echo '<label for="' .$this->get_field_id( 'fb_page_url' ). '">' ._e( 'Facebook Page URL:' ). '</label> ';
echo '<input class="widefat" id="'. $this->get_field_id( 'fb_page_url' ) .'" name="'. $this->get_field_name( 'fb_page_url' ) .'" type="text" value="'. esc_attr( $fb_page_url ) .'">';
echo '</p>';
//Facebook LikeBox Width
$fb_likebox_width = (isset($instance[ 'fb_likebox_width' ]))? $instance[ 'fb_likebox_width' ] : __( '300', 'text_domain' );
echo '<p>';
echo '<label for="' .$this->get_field_id( 'fb_likebox_width' ). '">' ._e( 'LikeBox Width:' ). '</label> ';
echo '<input class="widefat" id="'. $this->get_field_id( 'fb_likebox_width' ) .'" name="'. $this->get_field_name( 'fb_likebox_width' ) .'" type="text" value="'. esc_attr( $fb_likebox_width ) .'">';
echo '</p>';
//Facebook LikeBox Height
$fb_likebox_height = (isset($instance[ 'fb_likebox_height' ]))? $instance[ 'fb_likebox_height' ] : __( '300', 'text_domain' );
echo '<p>';
echo '<label for="' .$this->get_field_id( 'fb_likebox_height' ). '">' ._e( 'LikeBox Height:' ). '</label> ';
echo '<input class="widefat" id="'. $this->get_field_id( 'fb_likebox_height' ) .'" name="'. $this->get_field_name( 'fb_likebox_height' ) .'" type="text" value="'. esc_attr( $fb_likebox_height ) .'">';
echo '</p>';
//Facebook LikeBox Color Scheme
$fb_likebox_color = (isset($instance[ 'fb_likebox_color' ]))? $instance[ 'fb_likebox_color' ] : '';
echo '<p>';
echo '<label for="' .$this->get_field_id( 'fb_likebox_color' ). '">' ._e( 'LikeBox Color Scheme:' ). '</label> ';
echo '<select style="width: 100%;" id="'. $this->get_field_id( 'fb_likebox_color' ) .'" name="'. $this->get_field_name( 'fb_likebox_color' ) .'">';
echo '<option value="light">Light</option>';
if(esc_attr( $fb_likebox_color ) == "dark"){
echo '<option value="dark" selected>Dark</option>';
}else{
echo '<option value="dark">Dark</option>';
}
echo '</select>';
echo '</p>';
//Facebook Customization options
echo '<p>';
$ishow_face = ($instance[ 'ishow_face' ])? 'checked' : '';
$ishow_header = ($instance[ 'ishow_header' ])? 'checked' : '';
$ishow_posts = ($instance[ 'ishow_posts' ])? 'checked' : '';
$ishow_border = ($instance[ 'ishow_border' ])? 'checked' : '';
echo '<label>' ._e( 'Facebook Look:' ). '</label> ';
echo '<ul>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'ishow_face' ) .'" type="checkbox" value="1" '.$ishow_face.'>Show Friends\' Faces</li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'ishow_header' ) .'" type="checkbox" value="1" '.$ishow_header.'>Show Header</li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'ishow_posts' ) .'" type="checkbox" value="1" '.$ishow_posts.'>Show Posts</li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'ishow_border' ) .'" type="checkbox" value="1" '.$ishow_border.'> Show Border </li>';
echo '</ul>';
echo '</p>';
//Display Zones options
echo '<p>';
$ifront_page = ($instance[ 'ifront_page' ])? 'checked' : '';
$ipages = ($instance[ 'ipages' ])? 'checked' : '';
$itags = ($instance[ 'itags' ])? 'checked' : '';
$icats = ($instance[ 'icats' ])? 'checked' : '';
$iposts = ($instance[ 'iposts' ])? 'checked' : '';
echo '<label>' ._e( 'Display Zones:' ). '</label> ';
echo '<ul>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'ifront_page' ) .'" type="checkbox" value="1" '.$ifront_page.'>Front Page </li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'ipages' ) .'" type="checkbox" value="1" '.$ipages.'>Pages </li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'itags' ) .'" type="checkbox" value="1" '.$itags.'>Tagged Pages </li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'icats' ) .'" type="checkbox" value="1" '.$icats.'> Category Pages </li>';
echo '<li><input class="widefat" name="'. $this->get_field_name( 'iposts' ) .'" type="checkbox" value="1" '.$iposts.'> Posts </li>';
echo '</ul>';
echo '</p>';
}
//processes widget options to be saved
public function update( $new_instance, $old_instance ){
$instance = array();
//We simply add values to $instance array for saving.
$instance['title'] = ( ! empty( $new_instance['title'] ) ) ? strip_tags( $new_instance['title'] ) : '';
$instance['fb_page_url'] = ( ! empty( $new_instance['fb_page_url'] ) ) ? strip_tags( $new_instance['fb_page_url'] ) : '';
$instance['fb_likebox_width'] = ( ! empty( $new_instance['fb_likebox_width'] ) ) ? strip_tags( $new_instance['fb_likebox_width'] ) : '';
$instance['fb_likebox_height'] = ( ! empty( $new_instance['fb_likebox_height'] ) ) ? strip_tags( $new_instance['fb_likebox_height'] ) : '';
$instance['fb_likebox_color'] = ( ! empty( $new_instance['fb_likebox_color'] ) ) ? strip_tags( $new_instance['fb_likebox_color'] ) : '';
$instance['ishow_face'] = ( ! empty( $new_instance['ishow_face'] ) ) ? strip_tags( $new_instance['ishow_face'] ) : '';
$instance['ishow_header'] = ( ! empty( $new_instance['ishow_header'] ) ) ? strip_tags( $new_instance['ishow_header'] ) : '';
$instance['ishow_posts'] = ( ! empty( $new_instance['ishow_posts'] ) ) ? strip_tags( $new_instance['ishow_posts'] ) : '';
$instance['ishow_border'] = ( ! empty( $new_instance['ishow_border'] ) ) ? strip_tags( $new_instance['ishow_border'] ) : '';
$instance['ifront_page'] = ( ! empty( $new_instance['ifront_page'] ) ) ? strip_tags( $new_instance['ifront_page'] ) : '';
$instance['ipages'] = ( ! empty( $new_instance['ipages'] ) ) ? strip_tags( $new_instance['ipages'] ) : '';
$instance['itags'] = ( ! empty( $new_instance['itags'] ) ) ? strip_tags( $new_instance['itags'] ) : '';
$instance['icats'] = ( ! empty( $new_instance['icats'] ) ) ? strip_tags( $new_instance['icats'] ) : '';
$instance['iposts'] = ( ! empty( $new_instance['iposts'] ) ) ? strip_tags( $new_instance['iposts'] ) : '';
return $instance;
}
}
//register widget using the widgets_init
add_action( 'widgets_init', 'reg_facebook_likebox_widget' );
function reg_facebook_likebox_widget() {
register_widget( 'facebook_likebox_widget' );
}